In ExpressJS applications, environment variables play a crucial role in configuring sensitive information such as database credentials, API keys, and secret tokens. While storing these sensitive details directly in the source code is not recommended due to security concerns, keeping track of them separately can be cumbersome and error-prone.
This is where the dotenv package comes into play. dotenv is a lightweight Node.js library that facilitates the management of environment variables directly within the project directory. It allows you to store sensitive information securely outside the code, making it easier to maintain and update your application.
How to Use dotenv in ExpressJS
To integrate dotenv
into your ExpressJS application, follow these simple steps:
- Install the
dotenv
package using npm or yarn:npm install dotenv
- Create a
.env
file in the root directory of your project. This file will store your environment variables in key-value pairs. - Store environment variables: Add your sensitive information to the
.env
file, separating each key-value pair with an equal sign (=). For example:DATABASE_PASSWORD=your_password
API_KEY=your_api_key
- Load the environment variables from the
.env
file:const dotenv = require('dotenv');
Use thedotenv.config()
function to load the environment variables from the.env
file:
dotenv.config(); - Access environment variables: Now you can access your environment variables using the
process.env
object, like this:
const databasePassword = process.env.DATABASE_PASSWORD;
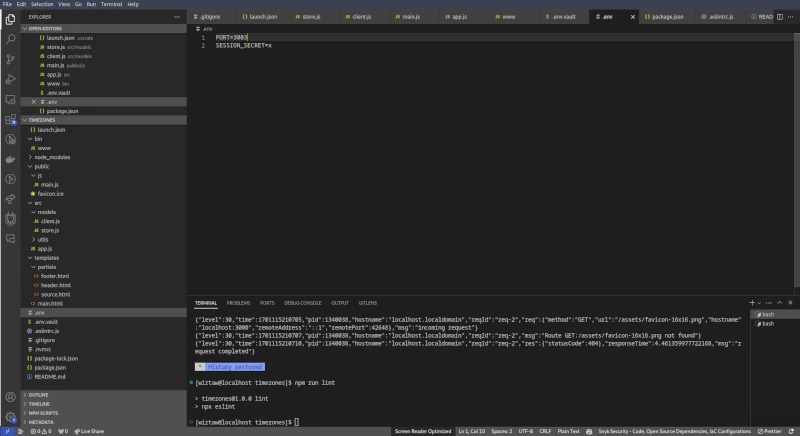

Benefits of Using dotenv
By using dotenv, you can enjoy several benefits:
- Secure storage: Sensitive information is stored in a separate file outside the code, preventing it from being exposed in the source code.
- Easy management: Environment variables can be easily modified and updated without changing the codebase.
- Consistency: Environment variables can be easily shared and used across different environments, such as development, staging, and production.
Now, you can access the environment variables using dotenv-provided methods, such as process.env.<VARIABLE_NAME>
. For example, to access the database password stored in the .env file:
const databasePassword = process.env.DATABASE_PASSWORD;
By using dotenv
, you can keep your environment variables secured and easily manage them outside the code, ensuring the security and maintainability of your ExpressJS application.
Conclusion
Incorporating dotenv
into your ExpressJS applications is a simple yet effective way to manage environment variables securely and efficiently. By following these steps, you can enhance the security and maintainability of your applications, keeping your sensitive information safe.
Leave a Reply