Add Unit Tests to Your ExpressJS Applications
Unit testing is a critical part of software development. It helps you to ensure that your code is working as expected and to catch bugs early on. Unit tests are especially important for ExpressJS applications, as they can help you to test your endpoints and controllers without having to deploy your application or rely on external services.
About Unit tests
To add unit tests to your ExpressJS application, you will need to install a unit testing framework. Jest is a popular choice for unit testing NodeJS applications. Jest is easy to use and provides a variety of features that make it ideal for unit testing, such as:
- Automatic test discovery: Jest will automatically discover and run your test files.
- Snapshot testing: Snapshot testing allows you to compare the output of your tests to a reference snapshot.
- Mock objects: Mock objects allow you to simulate the behavior of external dependencies.
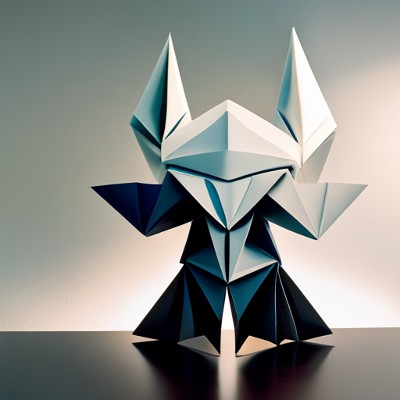
Add Jest and Supertest to ExpressJS application
To install Jest and Supertest, run the following command:
npm install jest supertest chai --save-dev
Once Jest is installed, you can start writing unit tests for your ExpressJS application. To create a new test file, create a file with the .test.js
or .spec.js
extension. For example, to create a test file for your users
controller, you would create a file called users.test.js
.
Chai is a popular assertion library for NodeJS and JavaScript testing frameworks. It provides a variety of assertion styles, including expect, assert, and should. Chai is also highly extensible, allowing you to create custom assertions and plugins.
In your test file, you will need to import the SuperTest
library. SuperTest is a library that allows you to make HTTP requests to your ExpressJS application. Import the expect
assertion style into your test file
const supertest = require('supertest');
const expect = require('chai').expect;
You will also need to import the ExpressJS application that you want to test.
const app = require('../app');
Once you have imported the necessary libraries, you can start writing unit tests for your ExpressJS endpoints. For example, the following test tests the GET /users
endpoint:
test('GET /users endpoint should return a list of users', async () => {
const response = await supertest(app).get('/users');
expect(response.status).toBe(200);
expect(response.body).toBeInstanceOf(Array);
});
To run your unit tests, simply run the following command:
npm run test
Conclusion
Jest will automatically discover and run all of your test files. If any of your tests fail, Jest will provide you with a detailed error message.
Adding unit tests to your ExpressJS application is a great way to ensure that your code is working as expected and to catch bugs early on. By following the steps above, you can easily add unit tests to your ExpressJS applications using Jest and SuperTest.
Testing JS page
Leave a Reply